mirror of
https://github.com/Significant-Gravitas/Auto-GPT.git
synced 2025-01-09 04:19:02 +08:00
Plugins initial
This commit is contained in:
parent
e62a3be294
commit
65b626c5e1
11
README.md
11
README.md
@ -204,6 +204,17 @@ export PINECONE_ENV="Your pinecone region" # something like: us-east4-gcp
|
||||
|
||||
```
|
||||
|
||||
## Plugins
|
||||
|
||||
See https://github.com/Torantulino/Auto-GPT-Plugin-Template for the template of the plugins.
|
||||
|
||||
WARNING: Review the code of any plugin you use.
|
||||
|
||||
Drop the repo's zipfile in the plugins folder.
|
||||
|
||||
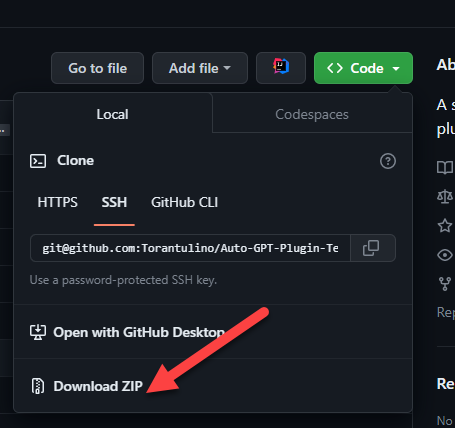
|
||||
|
||||
If you add the plugins class name to the whitelist in the config.py you will not be prompted otherwise you'll be warned before loading the plugin.
|
||||
|
||||
## View Memory Usage
|
||||
|
||||
|
BIN
plugin.png
Normal file
BIN
plugin.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 33 KiB |
0
plugins/__PUT_PLUGIN_ZIPS_HERE__
Normal file
0
plugins/__PUT_PLUGIN_ZIPS_HERE__
Normal file
@ -81,6 +81,10 @@ class Config(metaclass=Singleton):
|
||||
# Initialize the OpenAI API client
|
||||
openai.api_key = self.openai_api_key
|
||||
|
||||
self.plugins = []
|
||||
self.plugins_whitelist = []
|
||||
self.plugins_blacklist = []
|
||||
|
||||
def set_continuous_mode(self, value: bool):
|
||||
"""Set the continuous mode value."""
|
||||
self.continuous_mode = value
|
||||
@ -135,3 +139,7 @@ class Config(metaclass=Singleton):
|
||||
def set_debug_mode(self, value: bool):
|
||||
"""Set the debug mode value."""
|
||||
self.debug = value
|
||||
|
||||
def set_plugins(self, value: list):
|
||||
"""Set the plugins value."""
|
||||
self.plugins = value
|
||||
|
@ -22,5 +22,7 @@ def create_chat_completion(messages, model=None, temperature=None, max_tokens=No
|
||||
temperature=temperature,
|
||||
max_tokens=max_tokens
|
||||
)
|
||||
|
||||
return response.choices[0].message["content"]
|
||||
resp = response.choices[0].message["content"]
|
||||
for plugin in cfg.plugins:
|
||||
resp = plugin.on_response(resp)
|
||||
return resp
|
||||
|
@ -13,7 +13,10 @@ from enum import Enum, auto
|
||||
import sys
|
||||
from config import Config
|
||||
from json_parser import fix_and_parse_json
|
||||
from plugins import load_plugins
|
||||
from ai_config import AIConfig
|
||||
import os
|
||||
from pathlib import Path
|
||||
import traceback
|
||||
import yaml
|
||||
import argparse
|
||||
@ -323,6 +326,30 @@ user_input = "Determine which next command to use, and respond using the format
|
||||
memory = get_memory(cfg, init=True)
|
||||
print('Using memory of type: ' + memory.__class__.__name__)
|
||||
|
||||
|
||||
plugins_found = load_plugins(Path(os.getcwd()) / "plugins")
|
||||
loaded_plugins = []
|
||||
for plugin in plugins_found:
|
||||
if plugin.__name__ in cfg.plugins_blacklist:
|
||||
continue
|
||||
if plugin.__name__ in cfg.plugins_whitelist:
|
||||
loaded_plugins.append(plugin())
|
||||
else:
|
||||
ack = input(
|
||||
f"WARNNG Plugin {plugin.__name__} found. But not in the"
|
||||
" whitelist... Load? (y/n): "
|
||||
)
|
||||
if ack.lower() == "y":
|
||||
loaded_plugins.append(plugin())
|
||||
|
||||
if loaded_plugins:
|
||||
print(f"\nPlugins found: {len(loaded_plugins)}\n"
|
||||
"--------------------")
|
||||
for plugin in loaded_plugins:
|
||||
print(f"{plugin._name}: {plugin._version} - {plugin._description}")
|
||||
|
||||
cfg.set_plugins(loaded_plugins)
|
||||
|
||||
# Interaction Loop
|
||||
while True:
|
||||
# Send message to AI, get response
|
||||
|
74
scripts/plugins.py
Normal file
74
scripts/plugins.py
Normal file
@ -0,0 +1,74 @@
|
||||
"""Handles loading of plugins."""
|
||||
|
||||
from ast import Module
|
||||
import zipfile
|
||||
from pathlib import Path
|
||||
from zipimport import zipimporter
|
||||
from typing import List, Optional, Tuple
|
||||
|
||||
|
||||
def inspect_zip_for_module(zip_path: str, debug: bool = False) -> Optional[str]:
|
||||
"""
|
||||
Inspect a zipfile for a module.
|
||||
|
||||
Args:
|
||||
zip_path (str): Path to the zipfile.
|
||||
debug (bool, optional): Enable debug logging. Defaults to False.
|
||||
|
||||
Returns:
|
||||
Optional[str]: The name of the module if found, else None.
|
||||
"""
|
||||
with zipfile.ZipFile(zip_path, 'r') as zfile:
|
||||
for name in zfile.namelist():
|
||||
if name.endswith("__init__.py"):
|
||||
if debug:
|
||||
print(f"Found module '{name}' in the zipfile at: {name}")
|
||||
return name
|
||||
if debug:
|
||||
print(f"Module '__init__.py' not found in the zipfile @ {zip_path}.")
|
||||
return None
|
||||
|
||||
|
||||
def scan_plugins(plugins_path: Path, debug: bool = False) -> List[Tuple[str, Path]]:
|
||||
"""Scan the plugins directory for plugins.
|
||||
|
||||
Args:
|
||||
plugins_path (Path): Path to the plugins directory.
|
||||
|
||||
Returns:
|
||||
List[Path]: List of plugins.
|
||||
"""
|
||||
plugins = []
|
||||
for plugin in plugins_path.glob("*.zip"):
|
||||
if module := inspect_zip_for_module(str(plugin), debug):
|
||||
plugins.append((module, plugin))
|
||||
return plugins
|
||||
|
||||
|
||||
def load_plugins(plugins_path: Path, debug: bool = False) -> List[Module]:
|
||||
"""Load plugins from the plugins directory.
|
||||
|
||||
Args:
|
||||
plugins_path (Path): Path to the plugins directory.
|
||||
|
||||
Returns:
|
||||
List[Path]: List of plugins.
|
||||
"""
|
||||
plugins = scan_plugins(plugins_path)
|
||||
plugin_modules = []
|
||||
for module, plugin in plugins:
|
||||
plugin = Path(plugin)
|
||||
module = Path(module)
|
||||
if debug:
|
||||
print(f"Plugin: {plugin} Module: {module}")
|
||||
zipped_package = zipimporter(plugin)
|
||||
zipped_module = zipped_package.load_module(str(module.parent))
|
||||
for key in dir(zipped_module):
|
||||
if key.startswith("__"):
|
||||
continue
|
||||
a_module = getattr(zipped_module, key)
|
||||
a_keys = dir(a_module)
|
||||
if '_abc_impl' in a_keys and \
|
||||
a_module.__name__ != 'AutoGPTPluginTemplate':
|
||||
plugin_modules.append(a_module)
|
||||
return plugin_modules
|
Loading…
Reference in New Issue
Block a user